Article
How to Stop Text Overflow in React Native
January 6, 2025
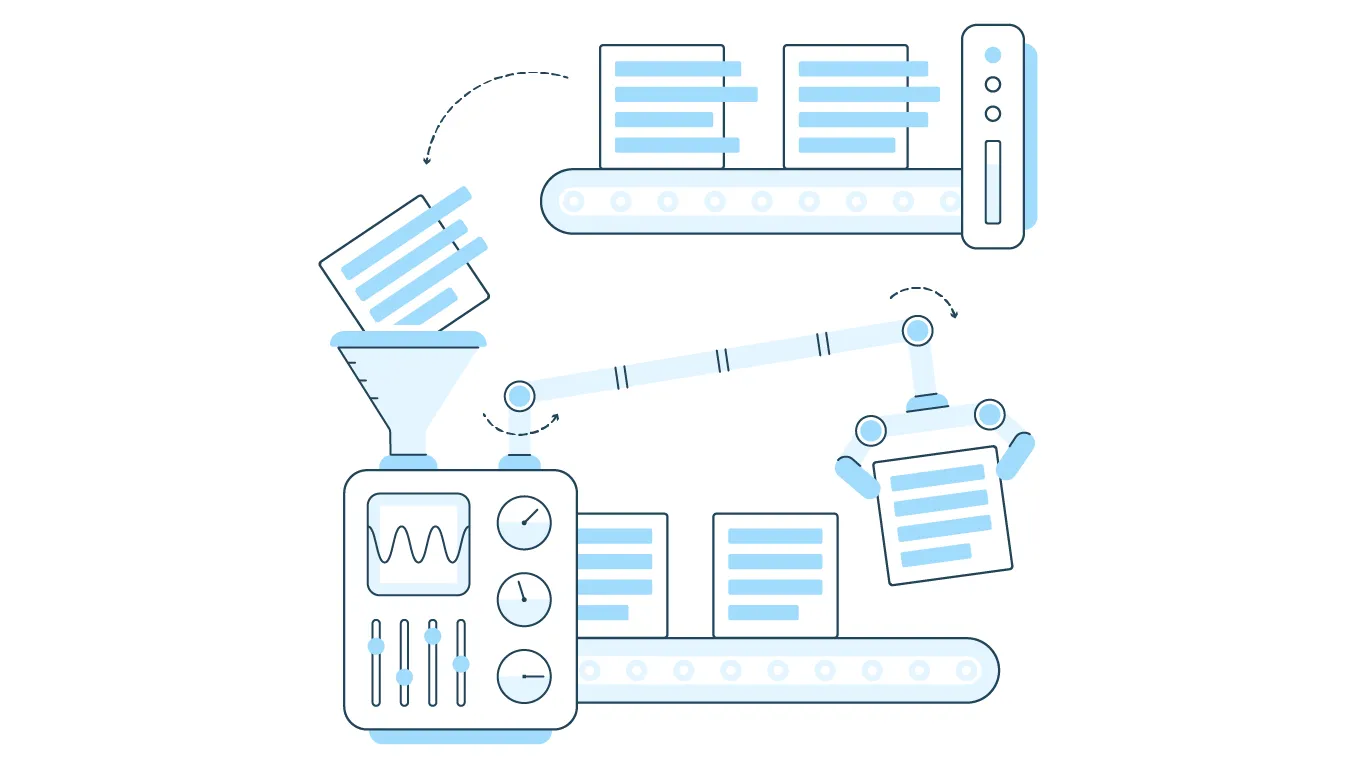
The Text
component is great at what it does, rendering text on screen, but it does have its own quirks. One of the biggest issues that I’ve run into is Text
not wrapping text at the correct width; instead it seems to ignore padding values when calculating the size that it needs to be, and will let the rendered text run out of the bounds of the component that it’s supposed to be contained within.
Thankfully, there’s a one-line fix for this: adjusting the layout behavior with flexShrink
.
Step 1: Find the text component that’s giving you a headache
In this simple to-do app, you can see that the text in the third to-do runs out of its own bounds.
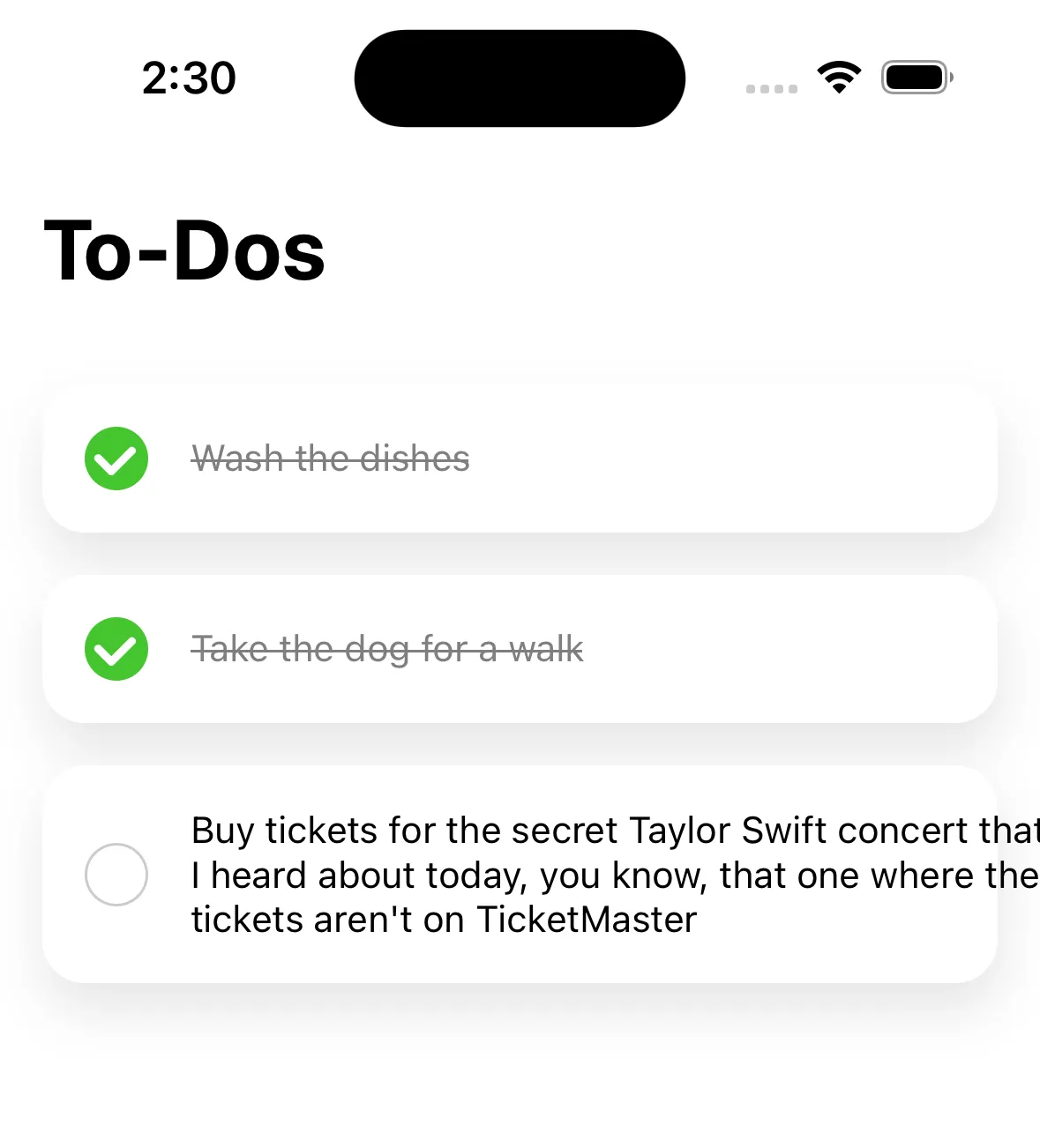
Step 2: Adjust flexShrink
Next, set the flexShrink
attribute in the Text
component’s style
to 1
. This instructs the React Native renderer to attempt to keep the component as small as possible within its parent’s bounds.
<Text
style={{
flexShrink: 1,
}}
>
Some really long text that should wrap to the next line… and not overflow the bounds like it did before?!?!
</Text>
And boom! Now your text component should listen to the padding applied to it and wrap the text as expected.
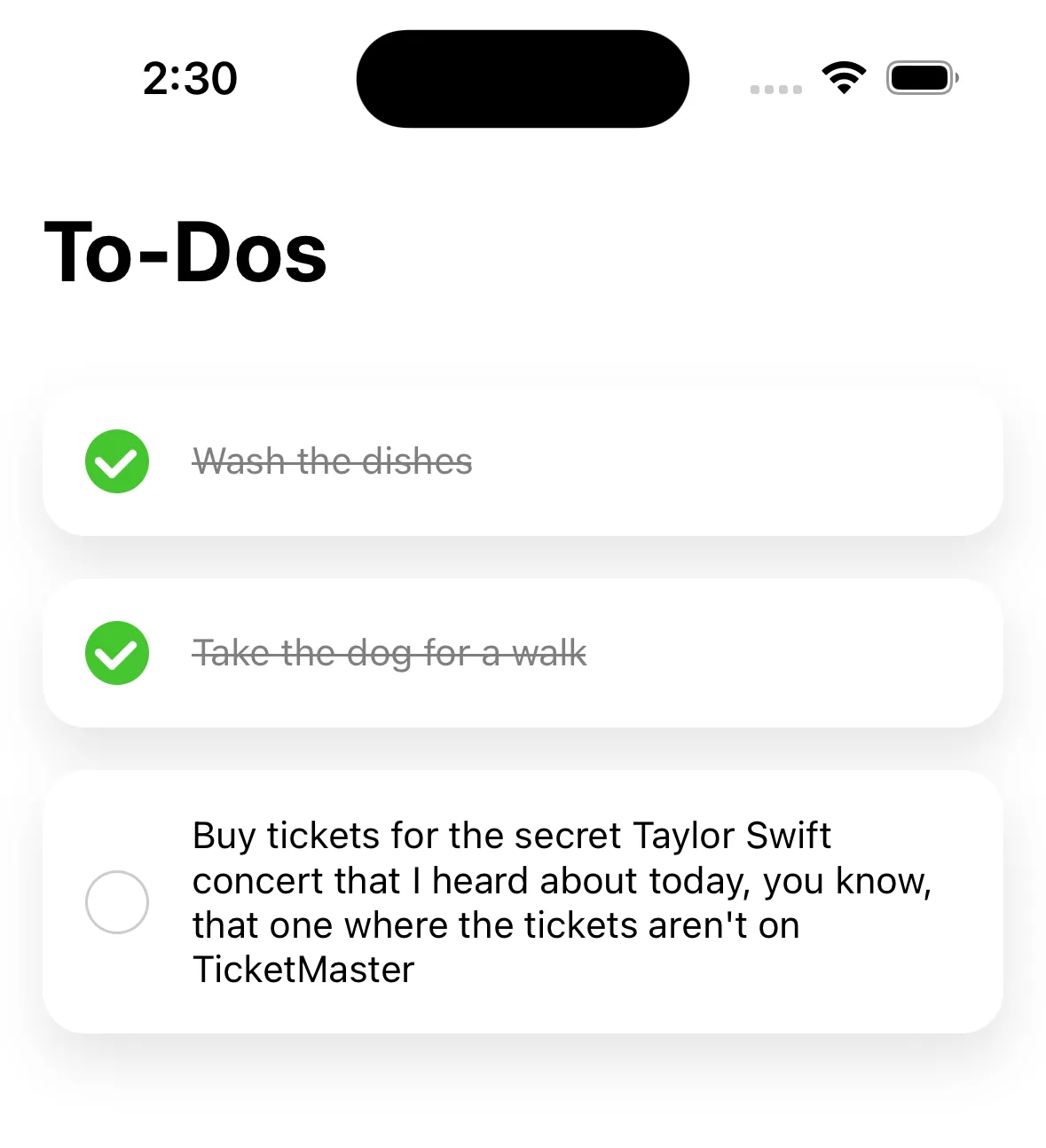
Other Solutions
When searching for a solution to this problem, I came across a few other solutions, including:
- Use
flexWrap: "wrap"
andflex: 1
on yourText
component: https://github.com/facebook/react-native/issues/1438#issuecomment-246743871 - Set
width: 0
and useflexGrow: 1
on yourText
component: https://github.com/facebook/react-native/issues/1438#issuecomment-278745825
While these solutions worked in specific use cases, I’ve found that the most reliable way to fix this issue is to use the flexShrink: 1
styling on your Text
component.